A Comprehensive Guide to Metaprogramming in Ru
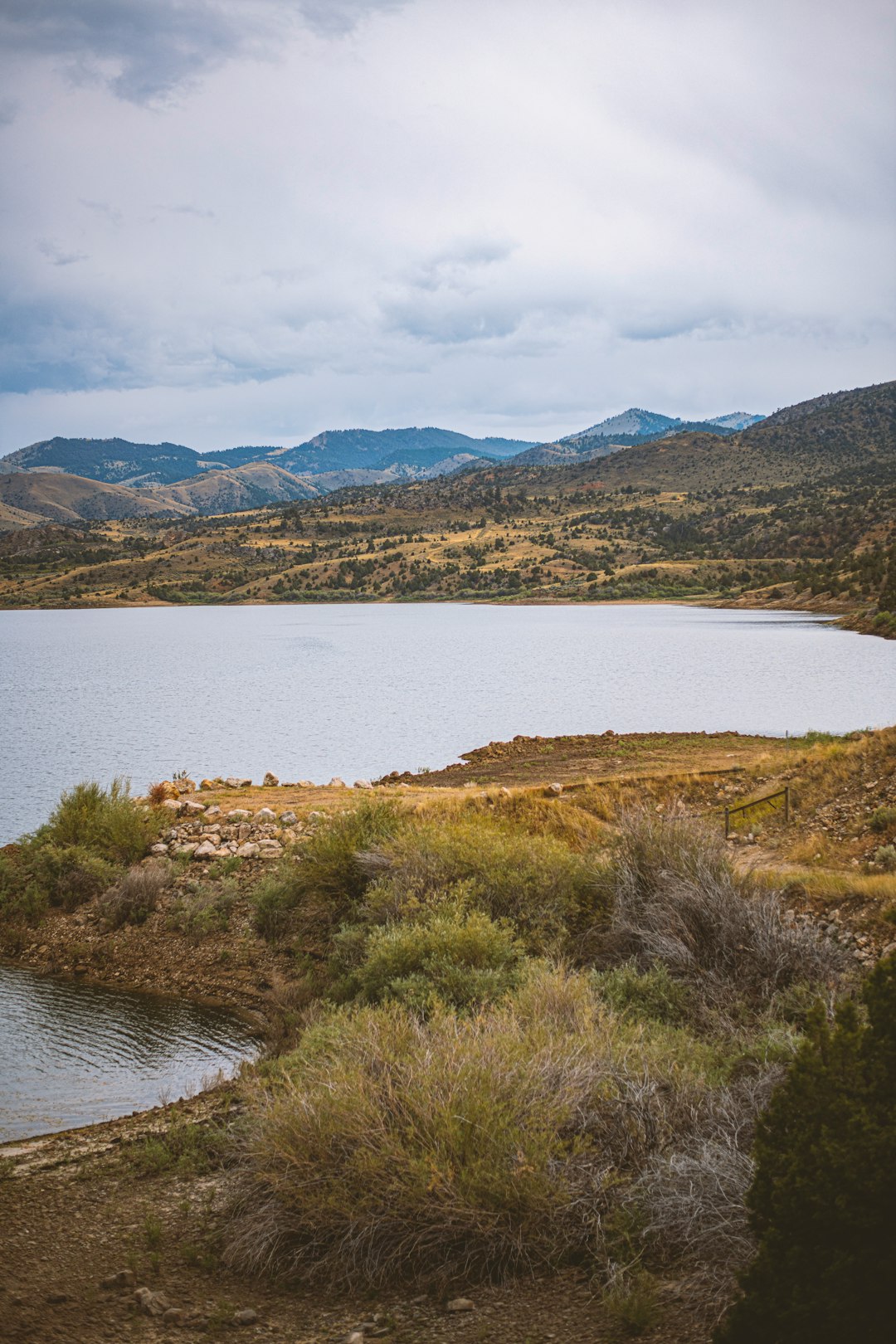
## Introduction
Metaprogramming is an advanced programming technique that allows you to manipulate the source code of a program at runtime. This enables the creation of dynamic and adaptable programs, as well as the capability to perform introspective operations. In Ruby, metaprogramming is particularly powerful due to its expressive and reflective nature.
## Concept Overview
Metaprogramming in Ruby involves the manipulation of objects that represent code. Central to this is the `metaclass`, a class that defines the behavior of a given class. By accessing a class's metaclass, you can modify its behavior, add new methods, or even create new classes on the fly. This level of control over the code itself makes metaprogramming a powerful tool for a variety of tasks.
## Detailed Explanation
Ruby's metaprogramming capabilities are built upon the concept of "self-representation." Every object in Ruby has a `class` method that returns its class, and every class has a `metaclass` method that returns its metaclass. This metaclass is responsible for defining the behavior of the class, such as what methods it can respond to and what its attributes are.
To access the metaclass, you can use either the `class << self` syntax or the `metaclass` method. Once you have access to the metaclass, you can modify its behavior using any of the standard object methods.
## Code Examples
```ruby
# Example 1: Adding a method to a class
class MyClass
class << self
def new_method
puts "Hello from the new method!"
end
end
end
MyClass.new_method # => "Hello from the new method!"
```
```ruby
# Example 2: Creating a new class on the fly
klass = Class.new do
def initialize(name)
@name = name
end
def greeting
"Hello, #{@name}!"
end
end
person = klass.new("John")
person.greeting # => "Hello, John!"
```
```ruby
# Example 3: Extending the String class
class String
def underscore
gsub(/::/, '/').
gsub(/([A-Z]+)([A-Z][a-z])/, '\1_\2').
gsub(/([a-z\d])([A-Z])/, '\1_\2').
tr("-", "_").
downcase
end
end
"MyCamelCaseString".underscore # => "my_camel_case_string"
```
## Common Pitfalls and Best Practices
One common pitfall when using metaprogramming is to modify the metaclass of a class that is already in use. This can lead to unexpected behavior, as the modified metaclass will apply to all instances of the class, even those that were created before the modification.
To avoid this, it is best practice to create a new class or module that inherits from the class you want to modify. This way, you can make changes to the metaclass without affecting existing instances of the original class.
## Advanced Applications
Metaprogramming can be used for a wide range of advanced applications, such as:
* Dynamic code generation: Creating new code at runtime, based on user input or other dynamic conditions.
* Introspection: Inspecting and modifying the behavior of a program at runtime.
* Dynamic DSLs: Creating domain-specific languages that can be extended and customized by users.
* Code optimization: Analyzing and modifying code to improve its performance.
## Conclusion
Metaprogramming in Ruby is a powerful technique that can unlock new possibilities for customization, flexibility, and extensibility in your code. By understanding the concepts and best practices outlined in this tutorial, you can harness the power of metaprogramming to create innovative and effective solutions in your Ruby applications.
Comments