Recursion – The Art of Self-Reference in Codi
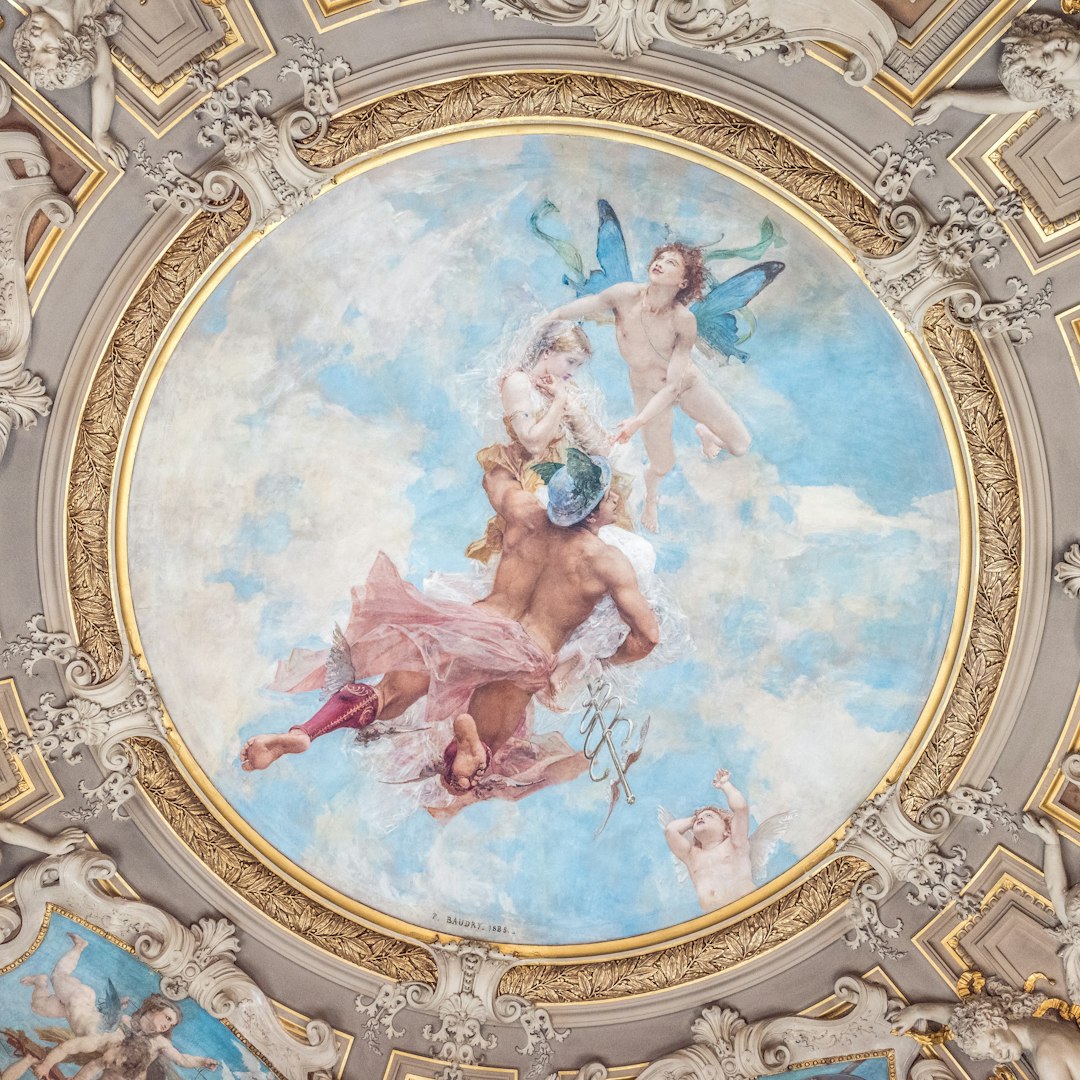
##
Introduction
Recursion, a technique of defining functions in terms of themselves, is a cornerstone of computing with far-reaching applications. It empowers programmers to tackle complex problems by breaking them down into smaller, recursive instances. By understanding this intriguing concept, developers can unlock a powerful tool for building efficient and elegant solutions.
##
Concept Overview
Recursion involves defining a function that can call itself to solve a problem. It relies on a base case, a condition that stops recursive calls and provides a specific result. The function then breaks the problem down into smaller subproblems, each of which is solved by another recursive call. This process continues until all subproblems are solved, and the original problem is resolved.
##
Detailed Explanation
Recursion works by manipulating the call stack, a data structure that keeps track of function calls. When a recursive function is called, a new entry is pushed onto the stack, which contains the parameters and local variables of that particular function call. The function then executes, and if it calls itself again, another entry is pushed onto the stack. This process continues until the base case is reached, at which point the stack entries start unwinding. Each entry pops from the stack, and the function returns its result to the caller, which uses it as its own output.
### Key Components:
- Base Case: A condition that stops the recursive calls and returns a specific value.
- Recursive Case: A situation where the function calls itself with different parameters to solve the problem.
- Tail Recursion: A special case where the recursive call is the last action executed in the function, resulting in increased efficiency.
##
Code Examples
```python
# 1. Factorial Calculation
def factorial(num):
if num == 0: # Base Case
return 1
else:
return num * factorial(num - 1) # Recursive Case
# 2. Fibonacci Sequence Generation
def fibonacci(num):
if num == 0 or num == 1: # Base Case
return 1
else:
return fibonacci(num - 1) + fibonacci(num - 2) # Recursive Case
# 3. Recursive Binary Search
def binary_search(arr, target, low, high):
if low > high: # Base Case - Target not found
return -1
else:
mid = (low + high) // 2
if arr[mid] == target: # Base Case - Target found
return mid
elif arr[mid] < target:
return binary_search(arr, target, mid + 1, high) # Recursive Case
else:
return binary_search(arr, target, low, mid - 1) # Recursive Case
# 4. Recursive Tower of Hanoi Puzzle
def tower_of_hanoi(n, from_rod, to_rod, aux_rod):
if n == 1:
return [[from_rod, to_rod]] # Base Case
else:
return tower_of_hanoi(n - 1, from_rod, aux_rod, to_rod) + [[from_rod, to_rod]] + tower_of_hanoi(n - 1, aux_rod, to_rod, from_rod)
```
##
Common Pitfalls and Best Practices
- Infinite Recursion: Ensure the base case is reached to avoid endless recursion.
- Stack Overflow: Monitor stack usage, as excessive recursion can lead to a stack overflow error.
- Unnatural Recursion: Avoid recursion if there are non-recursive alternatives that are more efficient or simpler to implement.
##
Advanced Applications
- Dynamic Programming: Recursion can be used to break down complex optimization problems into smaller subproblems, enabling the use of memoization techniques.
- Recursion Trees: Visualizing recursive calls as trees can help understand the flow of execution and identify potential performance issues.
- Divide-and-Conquer Algorithms: Recursion plays a central role in divide-and-conquer algorithms, which solve problems by dividing them into smaller parts, solving each part recursively, and combining the results.
##
Conclusion
Recursion is a powerful technique that allows programmers to solve complex problems elegantly and efficiently. By understanding the base case, recursive case, and key components, developers can harness the power of self-reference to create innovative and robust solutions. Whether it's calculating factorials, generating Fibonacci sequences, or solving puzzles like the Tower of Hanoi, recursion continues to be an indispensable tool in the programmer's arsenal. For further exploration, consider studying dynamic programming and recursion elimination techniques.
Comments