Understanding Currying: A Powerful Technique for Functional Programmi
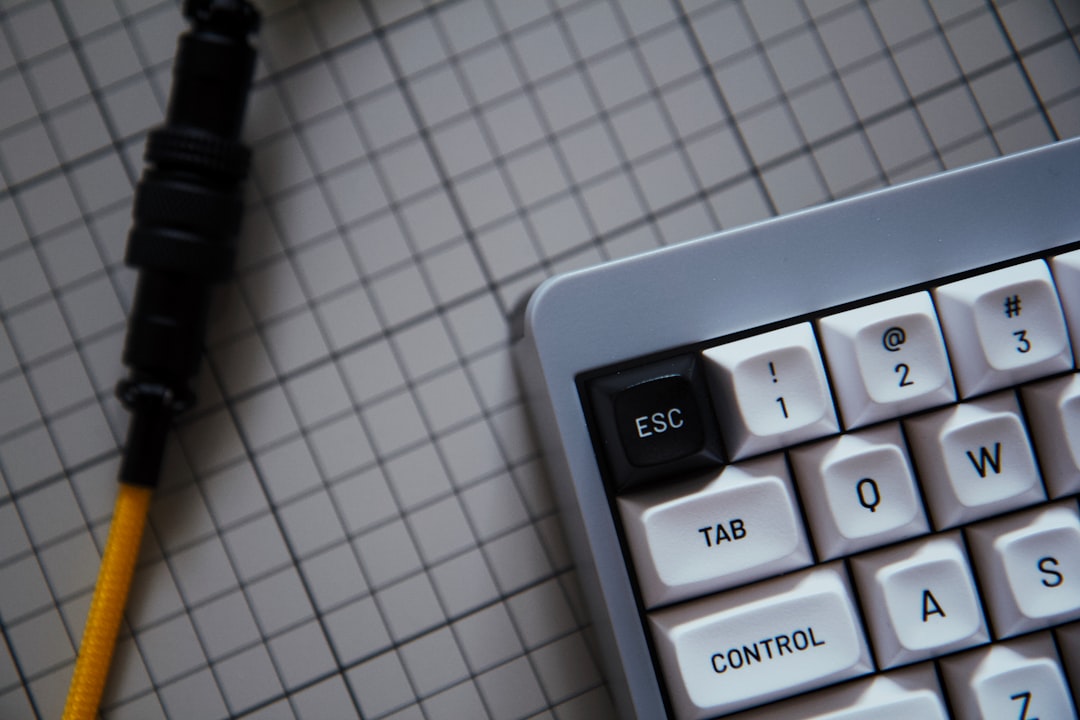
##
Introduction
Currying, a fascinating technique in functional programming, enables us to transform functions into smaller, reusable units. It introduces a unique approach to function application, empowering developers to create modular and composable programs. This tutorial will demystify the concept of currying, exploring its significance and how it can enhance your programming capabilities.
##
Concept Overview
Currying is a process of decomposing a multi-argument function into a series of single-argument functions. The resulting single-argument functions represent partial applications of the original function. By breaking down complex functions, currying allows for selective application of arguments, leading to greater flexibility and modularity.
##
Detailed Explanation
In its simplest form, a curried function takes one argument and returns a new function. This process continues until all arguments are consumed. For instance, a function f(x, y, z) can be curried as follows:
```
f_curried = f(x)
f_curried2 = f_curried(y)
result = f_curried2(z)
```
Currying allows us to partially apply arguments to a function. By fixing some arguments, we create new functions that can be reused or passed as parameters to other functions. This partial application enables us to build functions that can be easily composed and combined.
##
Code Examples
Example 1: Creating a curried function:
```python
def add(x, y):
return x + y
add_curried = curry(add) # Assume 'curry' is a function that creates a curried version of 'add'
```
Example 2: Partial application to calculate a specific sum:
```python
add_10 = add_curried(10)
result = add_10(5) # Equivalent to add(10, 5)
```
Example 3: Combining curried functions:
```python
def multiply(x, y):
return x * y
multiply_by_10 = curry(multiply)(10)
double = curry(multiply)(2)
result = multiply_by_10(double(5)) # Equivalent to multiply(10, multiply(2, 5))
```
Example 4: Using currying for concise code:
```python
def compose(f, g):
return lambda x: f(g(x))
add_10_and_double = compose(double, add_curried(10))
result = add_10_and_double(5) # Equivalent to double(add(10, 5))
```
##
Common Pitfalls and Best Practices
* Avoid over-currying: Currying can lead to excessive function nesting, making code difficult to read and maintain.
* Use descriptive function names: Well-named curried functions enhance readability and indicate their intended purpose.
* Leverage partial application: Partial functions allow for selective application of arguments, reducing the need for overloads.
##
Advanced Applications
* Memorization: Currying can be used to memoize functions, improving performance by storing and reusing previously computed results.
* Lazy evaluation: By deferring the evaluation of curried functions until necessary, we can optimize performance and avoid unnecessary calculations.
* Type checking: Currying enables type checking at each stage of function application, ensuring type safety and reducing errors.
##
Conclusion
Currying, a powerful technique in functional programming, enables the decomposition of functions into reusable units. By partially applying arguments, currying allows for greater modularity, composability, and concise code. Embracing currying empowers programmers to create elegant and efficient solutions, unlocking the full potential of functional programming. To delve deeper into currying, explore resources on functional programming and consider experimenting with different applications to master this valuable technique.
Comments