Unleashing the Power of Memoization: An Exploration for Enhanced Coding Performan
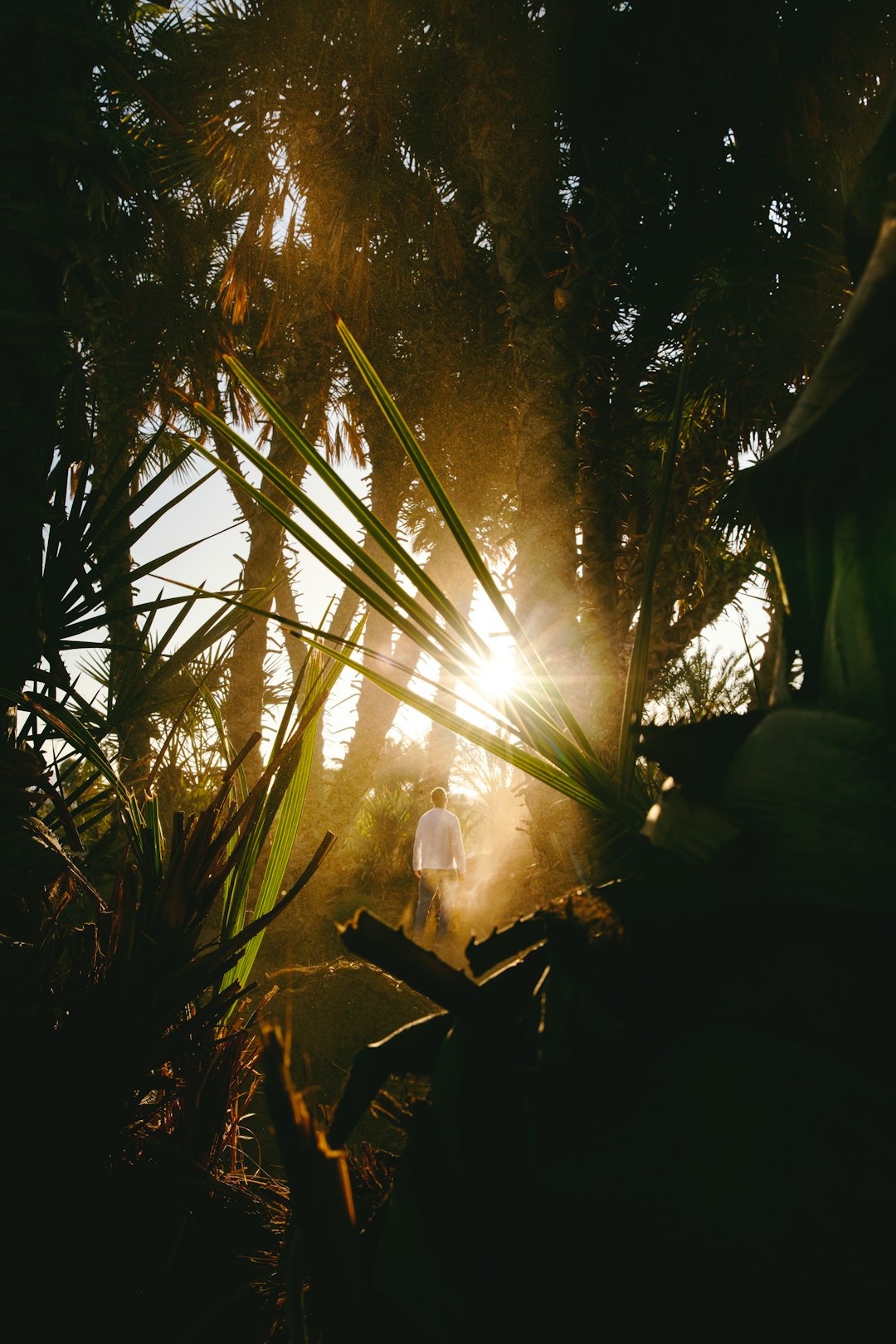
##
Introduction
In the fast-paced world of programming, optimizing code performance is paramount. Memoization, an ingenious technique that leverages caching to enhance code efficiency, has emerged as a game-changer. By caching the results of expensive calculations, memoization enables programs to bypass redundant computations, leading to significant speedups and improved memory utilization. Its impact extends far and wide, from web development to data processing, making it a highly valuable concept for both experienced and aspiring programmers.
##
Concept Overview
Memoization is a technique that stores the results of recursive function calls in a cache. When the function is called with the same arguments again, instead of recomputing the result, it retrieves the cached value, resulting in a significant reduction in execution time. This concept aligns perfectly with the dynamic programming paradigm, where overlapping subproblems are encountered frequently. By eliminating redundant calculations, memoization transforms exponential runtime complexities into linear ones, enhancing code efficiency substantially.
##
Detailed Explanation
The core of memoization lies in the cache, a data structure that stores the input-output pairs of function calls. When a function is invoked, its arguments are checked against the cache. If a match is found, the cached result is returned, avoiding the need for recalculation. However, if there is no match, the function proceeds with its usual execution, and the result is stored in the cache for future reference. This intelligent caching mechanism prevents the function from recomputing identical values, resulting in dramatic performance improvements.
##
Code Examples
```python
# Fibonacci series using memoization
cache = {}
def fib(n):
if n in cache:
return cache[n]
if n < 2:
return n
result = fib(n - 1) + fib(n - 2)
cache[n] = result
return result
```
```python
# Factorial calculation using memoization
memo = {0: 1}
def factorial(n):
if n in memo:
return memo[n]
if n == 0:
return 1
result = n * factorial(n - 1)
memo[n] = result
return result
```
##
Common Pitfalls and Best Practices
A common pitfall with memoization is forgetting to check the cache before performing the calculation. Always ensure that the cache is consulted before proceeding with the computation. Additionally, the choice of data structure for the cache is crucial. Hash tables or dictionaries excel in this role due to their efficient key-value lookup capabilities.
##
Advanced Applications
Memoization is not limited to recursive functions. It can be extended to dynamic programming problems, where subproblems overlap significantly. By memoizing the intermediate results, one can transform exponential-time algorithms into polynomial-time ones.
##
Conclusion
Memoization stands as a powerful technique for enhancing code performance and optimizing resource utilization. Its ability to cache and reuse computed results transforms complex algorithms into efficient ones. As programmers strive for better performance and scalability, understanding and leveraging memoization becomes essential. For those seeking further exploration, numerous resources and tutorials are available online to delve deeper into the intricacies of this intriguing concept.
Comments