Unveiling the Enigmatic World of Metaprogramming in Ru
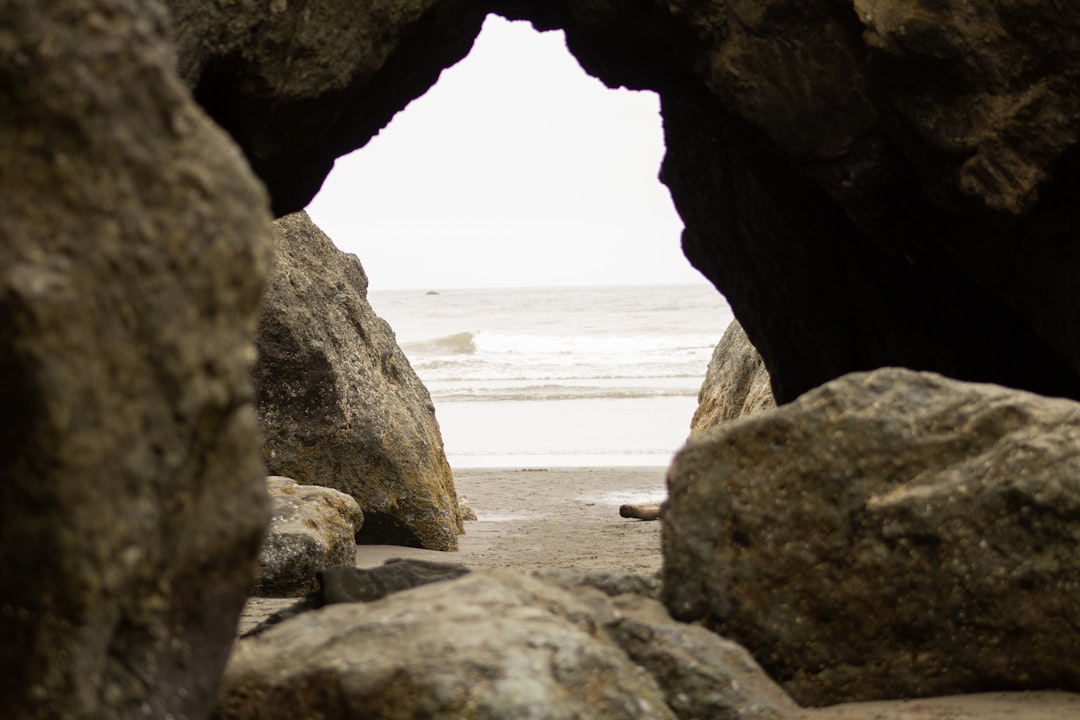
##
Introduction
Metaprogramming is a powerful programming technique that allows programmers to write code that manipulates and introspects other code. This transformative ability unlocks a world of possibilities, enabling the creation of self-modifying programs, dynamic type systems, and powerful abstractions. In the vibrant world of programming, metaprogramming is akin to a master architect, shaping the very foundations of code, empowering developers to craft sophisticated and extensible software.
##
Concept Overview
Metaprogramming, a fundamental concept in Ruby, extends beyond mere code execution. It empowers programmers to analyze, modify, and generate code dynamically during runtime. Programmers gain the ability to treat code as data, manipulating it like any other object. By leveraging Ruby's dynamic nature and powerful metaprogramming features like method_missing, define_method, and class_eval, programmers can mold their code to adapt to changing requirements, create custom DSLs, and build extensible frameworks.
##
Detailed Explanation
The heart of metaprogramming lies in the ability to dynamically access and modify a program's structure. Ruby's method_missing method allows objects to handle missing method calls, enabling programmers to intercept and respond to arbitrary method invocations. By dynamically defining new methods using define_method, programmers can extend classes and objects on the fly, adapting code to specific scenarios. class_eval, a potent tool, evaluates Ruby code within the context of a specific class or object, providing unparalleled flexibility in modifying class behavior at runtime.
##
Code Examples
1. Dynamically defining a method:
```ruby
class MyClass
def method_missing(method_name, *args, &block)
puts "Method #{method_name} called with args: #{args} and block: #{block}"
end
end
```
2. Extending a class with a new method:
```ruby
class Array
def shuffle!
self.sort_by { rand }
end
end
```
3. Modifying class behavior using class_eval:
```ruby
class MyClass
class_eval do
def greet
puts "Hello from MyClass"
end
end
end
```
##
Common Pitfalls and Best Practices
1. Avoid excessive metaprogramming, as it can lead to code that is difficult to understand and maintain.
2. Use metaprogramming thoughtfully and limit its scope to specific areas of your application.
3. Understand the performance implications of metaprogramming, as it can introduce runtime overhead.
##
Advanced Applications
Metaprogramming opens doors to advanced software engineering techniques. It empowers programmers to construct custom DSLs tailored to specific domains, enabling concise and expressive code. It also facilitates the creation of extensible frameworks where plugins and extensions can be dynamically loaded and integrated.
##
Conclusion
Metaprogramming is a powerful tool that empowers programmers to transcend the limitations of static code. By manipulating code as data, Ruby programmers can unlock a world of possibilities, ranging from dynamic type systems to self-modifying programs. However, it is crucial to approach metaprogramming with caution, ensuring its judicious use and careful consideration of its potential impact on code complexity and performance. By harnessing the power of metaprogramming, programmers can elevate their coding prowess and create truly remarkable software solutions.
Comments